Object-Oriented Programming (OOP) Meaning, Principles, Benefits.
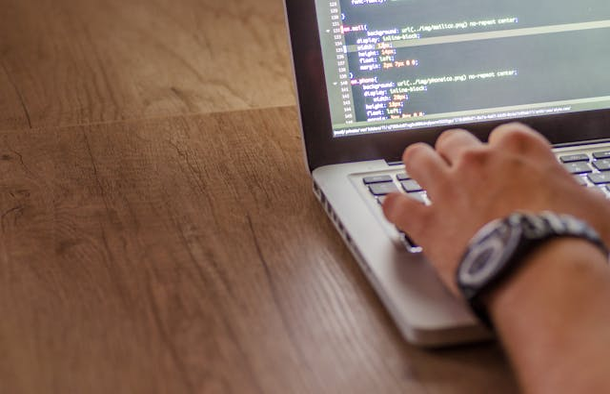
Object-oriented programming (OOP) is one of the most widely used programming paradigms in modern software development. It focuses on organizing code into reusable structures called objects, which represent real-world entities. These objects encapsulate data and behaviors, making code more modular, maintainable, and scalable.
At its core, OOP is based on the idea that software should be structured around objects rather than functions or procedures. This approach enables developers to create software systems that are more intuitive to understand, easier to manage, and adaptable to changes over time. Unlike procedural programming, where the focus is on step-by-step execution of functions, OOP encourages a more structured and reusable approach to coding.
Object-oriented programming plays a crucial role in various software development methodologies, including agile development, design patterns, and enterprise-level system architectures. By leveraging OOP principles, developers can create software that is robust, efficient, and easier to maintain, making it an essential skill for modern programmers.
The Four Core Principles of OOP: Encapsulation, Inheritance, Polymorphism, and Abstraction
Object-oriented programming is built on four foundational principles that define how objects interact with each other: encapsulation, inheritance, polymorphism, and abstraction. These principles provide a structured way to design software and ensure that code remains clean, efficient, and adaptable.
Encapsulation
Encapsulation refers to the practice of bundling data (variables) and methods (functions) into a single unit known as an object. This concept ensures that an object's internal state is hidden from outside interference, providing better security and data integrity. By restricting direct access to an object's data, encapsulation prevents unintended modifications and enhances maintainability. Access to data is typically controlled through getter and setter methods, allowing controlled interactions with an object.
Inheritance
Inheritance allows a class to acquire the properties and behaviors of another class. It enables code reuse and promotes hierarchical relationships between classes. Inheritance is particularly useful when building large applications, as it reduces redundancy by allowing new classes to inherit common attributes and methods from existing classes. This concept helps create a structured and scalable codebase where changes can be applied more efficiently.
Polymorphism
Polymorphism allows objects of different classes to be treated as objects of a common superclass. It enables a single interface to represent multiple underlying forms, providing flexibility in code design. Polymorphism is primarily achieved through method overloading (defining multiple methods with the same name but different parameters) and method overriding (modifying a method in a subclass). This principle is essential for designing flexible and scalable systems, as it allows developers to write generic and reusable code that can handle various object types dynamically.
Abstraction
Abstraction simplifies complex systems by exposing only the essential details while hiding the underlying implementation. It allows developers to focus on high-level concepts rather than getting bogged down by implementation details. Abstraction is typically implemented using abstract classes and interfaces, which define common behaviors without specifying exact implementations. This principle is especially useful in large-scale software development, where modularity and code organization are crucial.
Benefits of Using Object-Oriented Programming in Software Development
Object-oriented programming offers numerous advantages that make it a preferred choice for software development. Below are the key benefits, each explained in detail:
1. Code Reusability for Faster Development
One of the biggest advantages of OOP is the ability to reuse code, significantly reducing development time. Using inheritance, developers can create new classes based on existing ones, inheriting common functionalities without rewriting code from scratch. This eliminates redundancy, making applications more efficient and easier to scale.
2. Better Code Organization and Maintainability
OOP allows developers to structure their code logically, making it easier to manage and update over time. Since objects encapsulate both data and behavior, modifications can be made to specific parts of the program without affecting the entire system.
For instance, if a company needs to upgrade its user authentication system, they can modify the User class without altering the rest of the application. This makes maintenance more straightforward, reducing the risk of unintended errors when implementing changes.
3. Scalability for Large Applications
Modern software systems often require the ability to scale as they grow. OOP enables this by providing modularity, meaning developers can build applications with separate components that can be expanded or replaced without disrupting the entire system.
A banking system built using OOP can have independent modules for customer accounts, transactions, loans, and security features. If the bank decides to introduce a new loan type, they can add a new Loan class without modifying the existing system’s core functionalities.
4. Increased Security Through Encapsulation
Encapsulation ensures that an object’s internal state is protected from direct external modifications. Instead of allowing users to manipulate data freely, OOP provides controlled access using getter and setter methods, which ensures data integrity and prevents unauthorized changes.
For example, in an Employee class, sensitive details like salary or passwords can be kept private and accessed only through designated methods. This adds a layer of security, preventing accidental or malicious modifications of crucial data.
5. Easier Debugging and Troubleshooting
OOP makes debugging more manageable by isolating issues to specific objects or classes. Since each object is self-contained, developers can pinpoint errors without needing to scan the entire codebase.
For instance, if an application’s payment processing system malfunctions, developers can focus only on debugging the Payment class rather than checking every part of the program. This simplifies error tracking and accelerates the troubleshooting process.
6. Enhanced Collaboration Among Developers
In large development teams, OOP provides a structured way for multiple developers to work on the same project without interference. Since each class represents a distinct entity, team members can focus on individual components without overlapping their work.
7. Facilitates Use of Design Patterns
OOP enables developers to implement well-known design patterns, which are proven solutions to common software design problems. Patterns like Singleton, Factory, and Observer help streamline development, making applications more efficient and maintainable.
The Singleton pattern is used to ensure that only one instance of a class exists at a time, which is useful for managing database connections or logging systems in enterprise applications.
8. Flexibility and Adaptability
OOP makes applications highly adaptable to change, as objects can be modified, replaced, or extended without disrupting the entire system. This is particularly useful in industries where software evolves rapidly, such as finance, healthcare, and e-commerce.
Popular Programming Languages that Utilize OOP Concepts
Several programming languages support object-oriented programming principles, making OOP widely accessible to developers across various platforms.
- C++ – A powerful, high-performance language that supports both procedural and object-oriented programming. It is widely used in game development, system programming, and high-performance applications.
- Java – A fully object-oriented language known for its platform independence. Java is commonly used in enterprise applications, Android development, and large-scale systems.
- Python – A beginner-friendly language that fully supports OOP. Python’s simplicity and readability make it popular for web development, data science, and automation.
- C# – A language developed by Microsoft, extensively used for building Windows applications, game development with Unity, and enterprise software.
- Ruby – A dynamic and flexible language that follows OOP principles, often used for web development and scripting.
- Swift – Apple’s modern programming language designed for iOS and macOS development, with strong OOP support for building efficient and scalable applications.
Each of these languages implements OOP principles in unique ways, making them suitable for different types of applications and industries.
Common Design Patterns in Object-Oriented Programming
Design patterns are reusable solutions to common software development problems, helping developers build robust and maintainable applications. Some of the most widely used design patterns in OOP include:
- Singleton Pattern– Ensures that only one instance of a class exists throughout an application. Commonly used in database connections and logging mechanisms.
- Factory Pattern – Provides a way to create objects without specifying the exact class. It helps in managing object creation efficiently and is widely used in frameworks and libraries.
- Observer Pattern – Defines a one-to-many dependency between objects, ensuring that changes in one object trigger updates in its dependents. Used in event-driven programming and UI frameworks.
These design patterns enhance software architecture by promoting code organization, scalability, and maintainability.
Tips for Transitioning from Procedural to Object-Oriented Programming Mindset
For developers accustomed to procedural programming, shifting to an object-oriented mindset can be challenging. However, understanding the core differences and practicing OOP concepts can ease the transition.
One of the first steps is to start thinking in terms of objects rather than functions. Instead of writing procedural code that processes data, developers should focus on designing objects that encapsulate both data and behavior.
Using real-world analogies can help grasp OOP concepts more effectively. For instance, treating a "Car" as an object with attributes like "color" and "model" and behaviors like "drive" and "brake" makes OOP easier to understand.
Practicing with small projects and exercises can also accelerate learning. Many online resources provide tutorials and coding challenges to help beginners develop an OOP mindset.
Conclusion
Object-oriented programming is a fundamental approach to modern software development that enhances code organization, reusability, and scalability. Mastering OOP principles like encapsulation, inheritance, polymorphism, and abstraction, will help developers build efficient and maintainable software systems. While working with Java, Python, C++, or other OOP-based languages, embracing object-oriented programming unlocks new possibilities for writing cleaner, more structured, and professional code.Want to Build Something Amazing?
We prioritize your business success and we deliver faster. Our services are custom and build for scale.
Start nowMore Articles
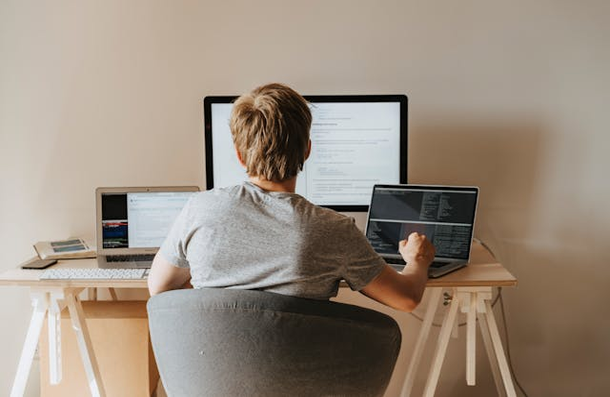
How to fix Common Database Design Errors and Improve Performance
23 hours, 17 minutes ago · 17 min read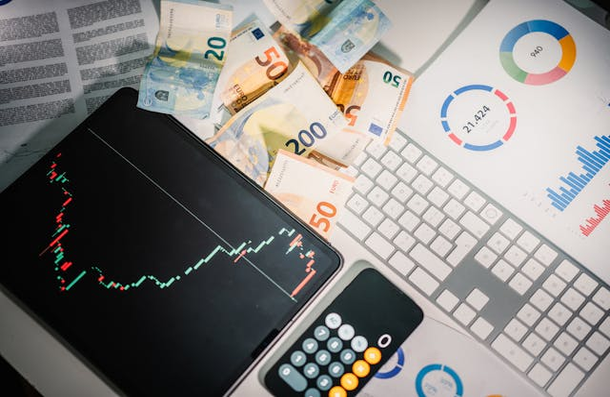
Making Money with Domain Names: A Guide to Buying and Selling Premium Domains
1 week, 1 day ago · 10 min read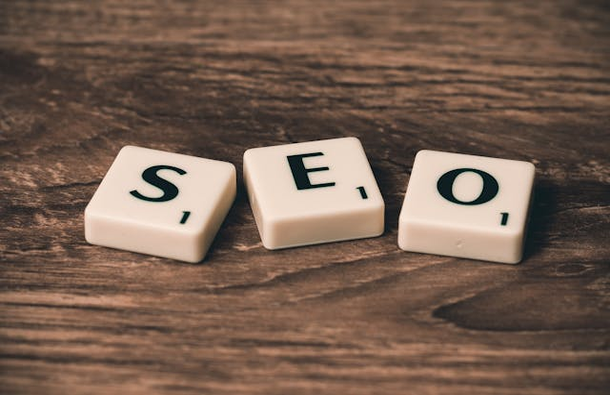
Why local SEO is Important for your Business Website
1 week, 4 days ago · 10 min read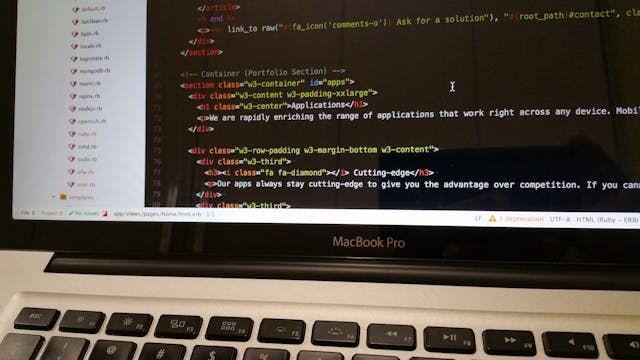
JavaScript for web development and programming. (Importance, Features, Roles of JavaScript in web development)
1 week, 4 days ago · 8 min read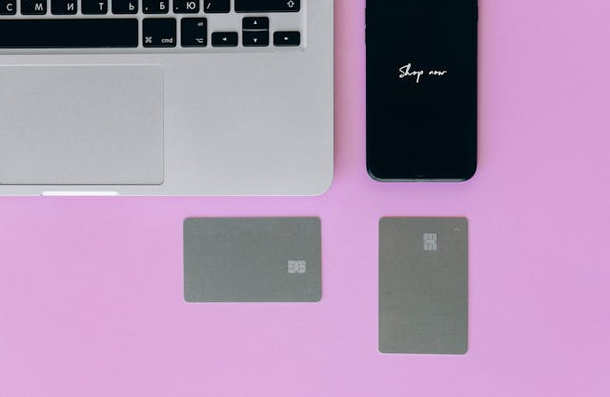
How to Integrate Payment Gateways into a Website ?
2 weeks, 4 days ago · 11 min read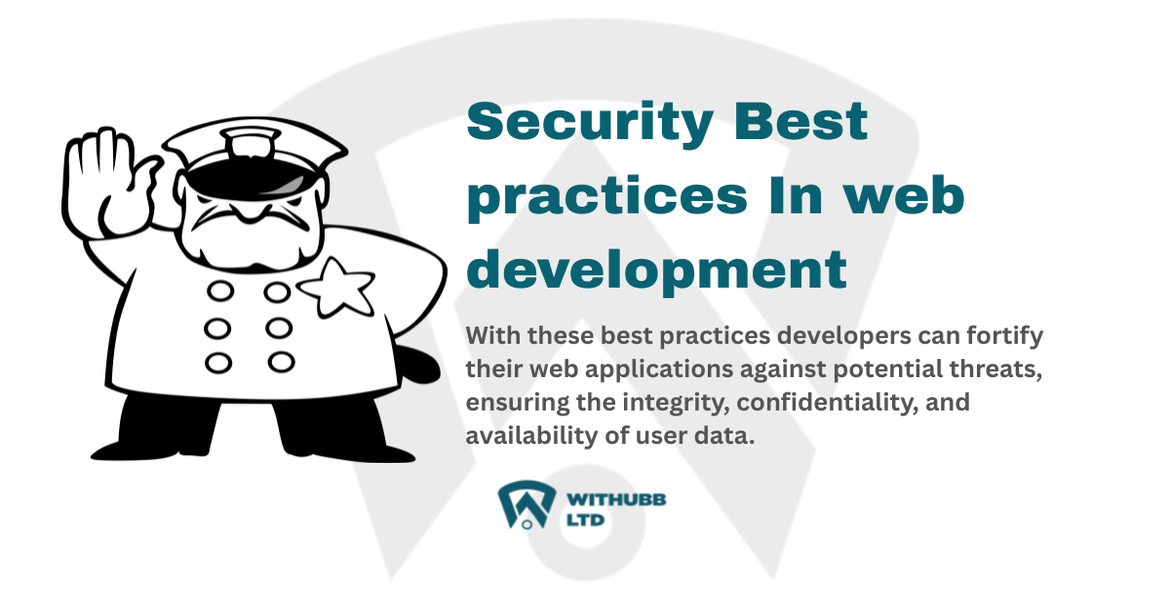
Security Best practices In web development
1 month, 1 week ago · 9 min read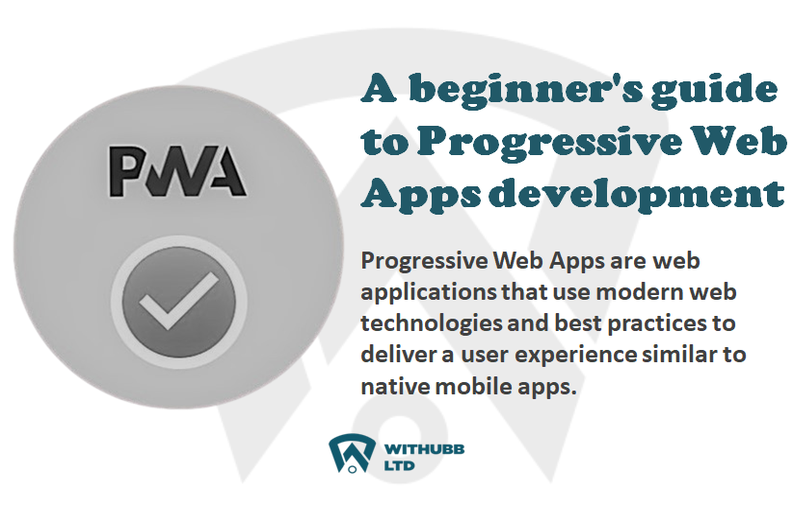
A beginner's guide to Progressive Web Apps development (PWA)
1 month, 1 week ago · 5 min read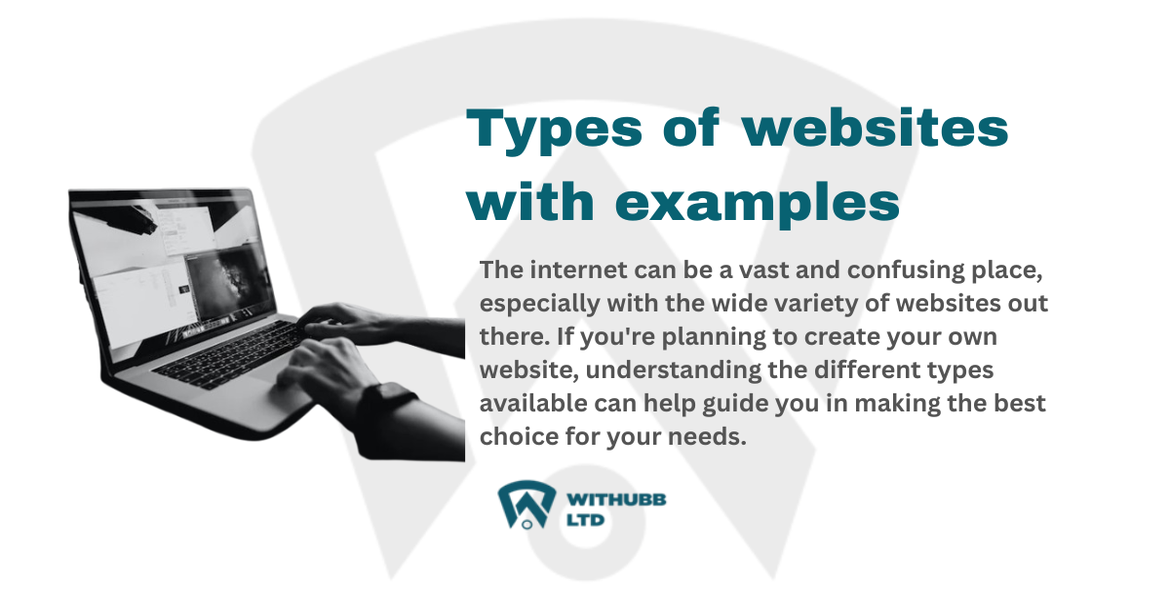
Types of websites with examples
1 month, 1 week ago · 10 min read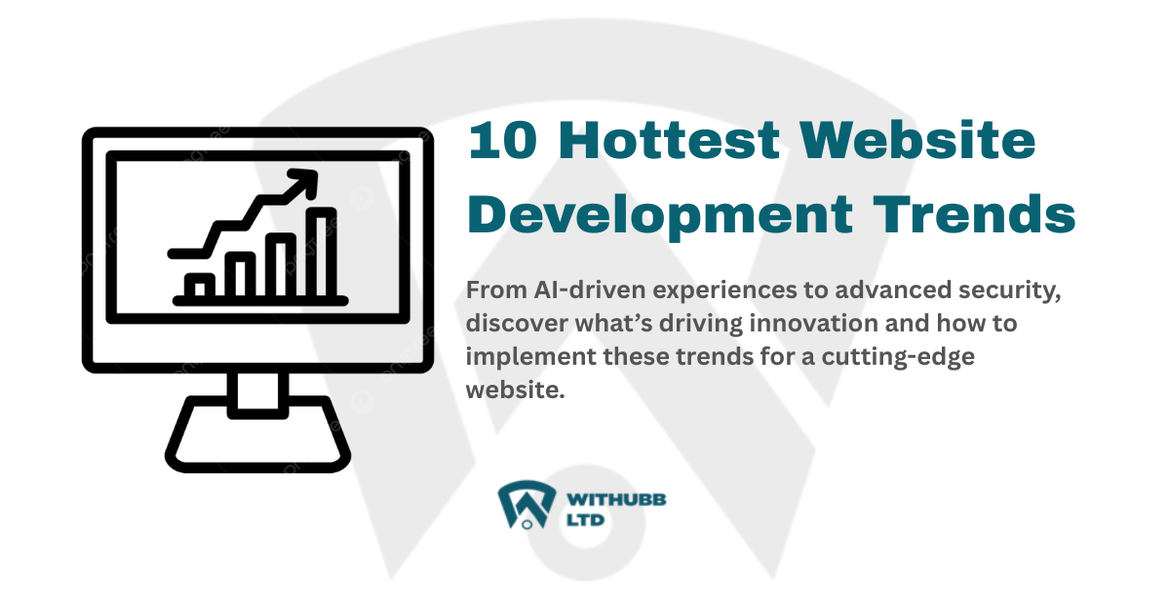
10 Hottest Website Development Trends You Can’t Ignore in 2025
1 month, 1 week ago · 10 min read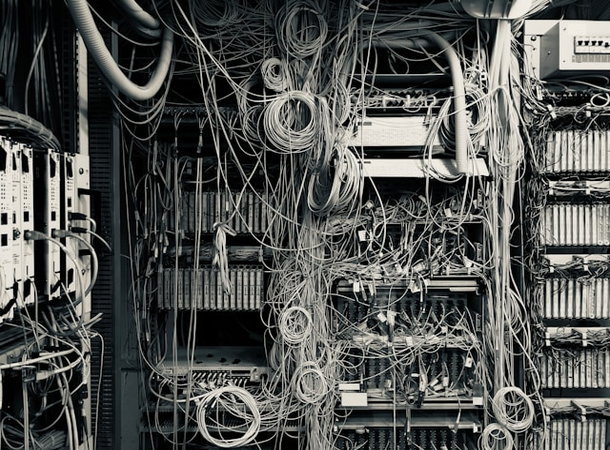
How to Set Up Django with PostgreSQL, Nginx, and Gunicorn on Ubuntu VPS Server
1 month, 2 weeks ago · 12 min read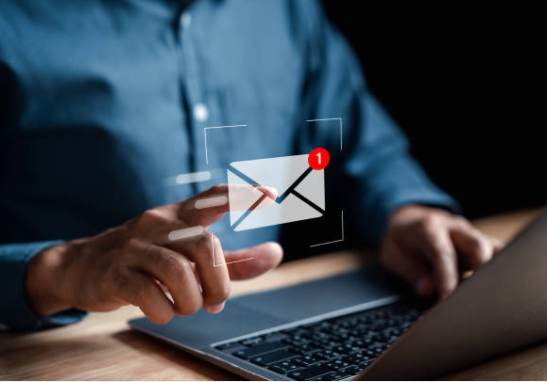
x