SQL Queries: A comprehensive guide for beginners
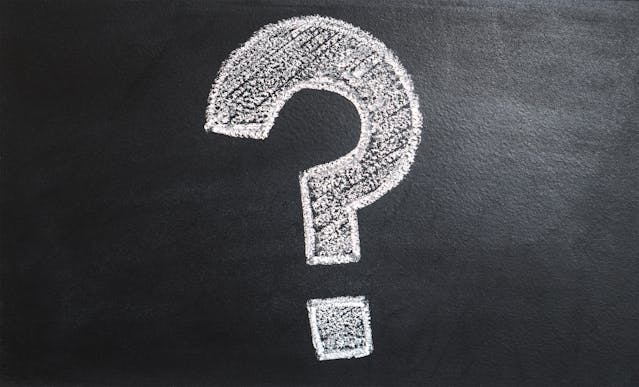
Introduction
Structured Query Language (SQL) is the foundation of database management, allowing users to retrieve, store, update, and delete data efficiently.
For beginners, SQL may seem complex at first, but once you understand the structure and logic behind it, writing queries becomes second nature. SQL is widely used across industries, from e-commerce platforms managing customer transactions to financial institutions handling vast amounts of data.
This guide is designed to take you from the absolute basics to more advanced concepts, ensuring you develop a strong grasp of SQL syntax, best practices, and real-world applications. By the end of this guide, you’ll be confident in writing SQL queries, retrieving meaningful data, optimizing performance, and managing databases effectively.
Understanding SQL and Relational Databases
What is SQL?
SQL (Structured Query Language) is a powerful language used to interact with relational databases. It is used for managing and manipulating structured data in relational databases. SQL is essential in software development, data analysis, and managing business information systems. It allows users to perform a variety of tasks, including:
- Create and retrieve data efficiently.
- Modifying existing records.
- Defining and managing database structures.
- Controlling access and security within a database.
SQL is the backbone of modern data-driven applications, enabling organizations to handle large amounts of structured data with accuracy and efficiency. Unlike programming languages like Python or Java, SQL is declarative, meaning you specify what you want the database to do, and the database engine determines how to execute the request.
What is a Relational Database?
A relational database organizes data into structured tables that relate to each other through key identifiers. This approach ensures data consistency, eliminates redundancy, and improves query efficiency. Relational databases store information in a tabular format, where:
- Tables contain data organized into rows and columns.
- Rows (Records) represent individual entries within a table.
- Columns (Fields) define attributes of the stored data, such as names, dates, or numerical values.
- Keys establish relationships between tables, ensuring data integrity.
- Primary Keys Unique identifiers for each record (e.g., id).
- Foreign Keys References that connect tables (e.g., customer_id in an Orders table referring to id in a Customers table).
For example, in an e-commerce platform, a relational database may include:
- A Customers table storing personal details like name, email, and address.
- An Orders table tracking purchases made by each customer.
- A Products table containing product information/ information on available items.
By using primary keys (unique identifiers for each row) and foreign keys (references to primary keys in related tables), relational databases efficiently manage connections between different data points.
How Do Databases Work?
A database is an organized collection of structured data, stored electronically in a way that allows efficient access and management. Think of it as a virtual filing cabinet where data is stored in tables, similar to spreadsheets.
Each database consists of:
Tables: The primary structure where data is stored. Each table is designed with a clear schema that defines the type of data it holds.
Schemas: Blueprints for tables, outlining field names, data types, and constraints.
Indexes: Special data structures that improve query speed by allowing the database engine to locate data faster.
Queries: SQL commands that retrieve, update, or delete data based on specific conditions.
SQL enables users to efficiently query data, filter results, and perform operations with minimal complexity. Now, let’s explore the essential SQL queries that serve as the foundation of database management.
SQL Query Basics
SQL queries follow a structured syntax. Let’s start with the fundamental commands every beginner must learn.
1. Retrieving Data with SELECT
The SELECT
statement is the most fundamental SQL command, allowing users to extract specific information from a table.
Basic Syntax
```sql
SELECT column_name FROM table_name;
To select all columns, use *:
```sql
SELECT * FROM table_name;
Example
If we have a `customers` table:
| id | name | age | city |
|----|-------|-----|----------|
| 1 | John | 30 | New York |
| 2 | Sarah | 25 | Chicago |
| 3 | Mike | 35 | Miami |
To retrieve all customers:
```sql
SELECT * FROM customers;
To retrieve only names and ages:
sql
SELECT name, age FROM customers;
2. Filtering Data with WHERE
The WHERE clause filters results ensuring you retrieve only relevant data.
ExampleRetrieve customers older than 30:
```sql
SELECT * FROM customers WHERE age > 30;
Retrieve customers from Chicago:
```sql
SELECT * FROM customers WHERE city = 'Chicago';
Common Operators used in
WHERE:
- - = (Equal)
- - != or <> (Not equal)
- - > (Greater than)
- - < (Less than)
- - >= (Greater than or equal to)
- - <= (Less than or equal to)
- - LIKE (Pattern matching)
- - IN (Match multiple values)
- - BETWEEN (Select a range)
3. Sorting Data with ORDER BY
Sort query results in ascending (`ASC`) or descending (`DESC`) order.
ExampleSort customers by age in ascending order:
```sql
SELECT * FROM customers ORDER BY age ASC;
Sort customers by name in descending order:
```sql
SELECT * FROM customers ORDER BY name DESC;
4. Limiting Results with LIMIT
Retrieve a specific number of records from a table.
ExampleGet only the first two customers:
```sql
SELECT * FROM customers LIMIT 2;
Working with Multiple Tables (Joins in SQL)
In real-world applications, data is rarely stored in a single table. Instead, databases use relationships to link multiple tables efficiently. SQL provides various types of joins to combine data from different tables.
Types of SQL Joins
Joins allow data from multiple tables to be combined based on related columns.
1. INNER JOIN: Returns matching records from both tables.
2. LEFT JOIN: Returns all records from the left table and matching records from the right table.
3. RIGHT JOIN: Returns all records from the right table and matching records from the left table.
4. FULL JOIN: Returns all records when there is a match in either table.
1. INNER JOIN
Returns only matching records from both tables.
ExampleRetrieve order details with customer names:
```sql
SELECT orders.order_id, customers.name
FROM orders
INNER JOIN customers ON orders.customer_id = customers.id;
2. LEFT JOIN and RIGHT JOIN
LEFT JOIN: Returns all records from the left table and matching records from the right table.
RIGHT JOIN: Returns all records from the right table and matching records from the left table.
Example```sql
SELECT customers.name, orders.order_id
FROM customers
LEFT JOIN orders ON customers.id = orders.customer_id;
Modifying Data with SQL Queries
1. Inserting Data with INSERT INTO
Add new records.
Example```sql
INSERT INTO customers (name, age, city) VALUES ('Emily', 28, 'Los Angeles');
2. Updating Data with UPDATE
Modify existing records.
Example```sql
UPDATE customers SET city = 'San Francisco' WHERE name = 'Emily';
3. Deleting Data with DELETE
Remove records from a table.
Example```sql
DELETE FROM customers WHERE age < 25;
Advanced SQL Concepts for Mastery
SQL provides built-in functions that allow users to perform calculations, modify text, and analyze data more effectively. These functions make it easier to extract insights, summarize information, and enhance query results.
1. Aggregate Functions
Aggregate functions perform calculations on a set of values and return a single result. These functions are commonly used in reports, summaries, and statistical analysis.
- COUNT() – Counts records
- SUM() –Adds up values in a specified column.
- AVG() – Calculates the average of a column’s values.
- MAX() / MIN() – Finds highest and lowest values
Example
Find the total number of customers:
```sql
SELECT COUNT(*) FROM customers;
Find the average age of customers: ```sql
SELECT AVG(age) FROM customers;
2. Date and Time Functions
Date functions allow you to work with timestamps, extract specific components from a date, and perform calculations based on time.
NOW(): Returns the current date and time.
CURDATE(): Returns the current date.
YEAR(), MONTH(), DAY(): Extracts specific parts of a date.
DATEDIFF(): Calculates the difference between two dates.
Example: Extracting Year and Month
Retrieve the year when customers registered:
SELECT name, YEAR(registration_date) FROM customers;
Find the number of days since an order was placed:
```sql
SELECT DATEDIFF(NOW(), order_date) FROM
3. Subqueries
A subquery is a query nested within another query, often used for filtering results dynamically. In other words. A query inside another query.
Example: Using a Subquery in WHERE ClauseFind customers older than the average age:
```sql SELECT * FROM customers WHERE age > (SELECT AVG(age) FROM customers);
4.. Indexing for Performance Optimization
Indexes speed up searches by allowing the database to locate rows faster. However, excessive indexing can slow down insert/update operations.
Example```sql
CREATE INDEX idx_customer_name ON customers (name);
5. Views
A view is a virtual table that stores a SQL query as a reusable object. Views help simplify complex queries and enhance security by restricting direct access to tables.
Example: Creating a View```sql
CREATE VIEW high_value_orders AS
SELECT customers.name, orders.total_price
FROM customers
INNER JOIN orders ON customers.id = orders.customer_id
WHERE orders.total_price > 200;
Now, you can retrieve high-value orders easily:
SELECT * FROM high_value_orders;
Common SQL Mistakes to Avoid
- Forgetting WHERE in UPDATE/DELETE: This can modify or delete all records unintentionally.
- Using SELECT * Carelessly: Always specify columns for efficiency.
- Ignoring Indexing: Large datasets run slower without proper indexing.
- Not Normalizing Data: Poor table design leads to redundancy and inefficiency.
Best Practices for Writing Efficient SQL Queries
- Write readable queries. Use proper formatting and indentation.
- Use proper indexing to optimize queries.
- Avoid redundant data retrieval by selecting only necessary columns.
- Normalize database design to reduce redundancy.
- Use joins instead of subqueries when possible for better performance.
- Always test queries on a small dataset before running on large databases.
Key Takeaways
- SQL is the foundation of modern data management, enabling users to efficiently interact with relational databases.
- Understanding relational databases is crucial for working with structured data, as they store information in connected tables.
- Basic SQL commands like SELECT, WHERE, ORDER BY, and LIMIT are essential for querying and retrieving data effectively.
- Joins allow users to combine data from multiple tables, making queries more powerful and insightful.
- Advanced SQL features like aggregate functions, subqueries, and indexing enhance query performance and data analysis.
- Best practices in SQL include writing efficient queries, avoiding redundant data retrieval, and ensuring database optimization.
Mastering SQL is a valuable skill, opening doors to data analysis, software development, and database administration. As you continue to explore SQL, practice writing queries, experiment with real datasets, and apply best practices to optimize performance. The more hands-on experience you gain, the more confident you’ll become in handling complex data operations.
Want to Build Something Amazing?
We prioritize your business success and we deliver faster. Our services are custom and build for scale.
Start nowMore Articles
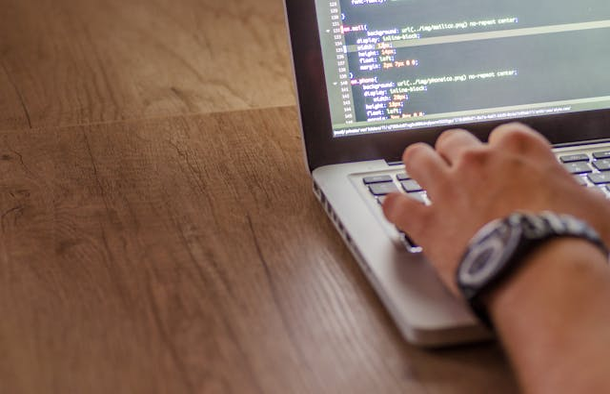
Object-Oriented Programming (OOP) Meaning, Principles, Benefits.
1 week, 1 day ago · 7 min read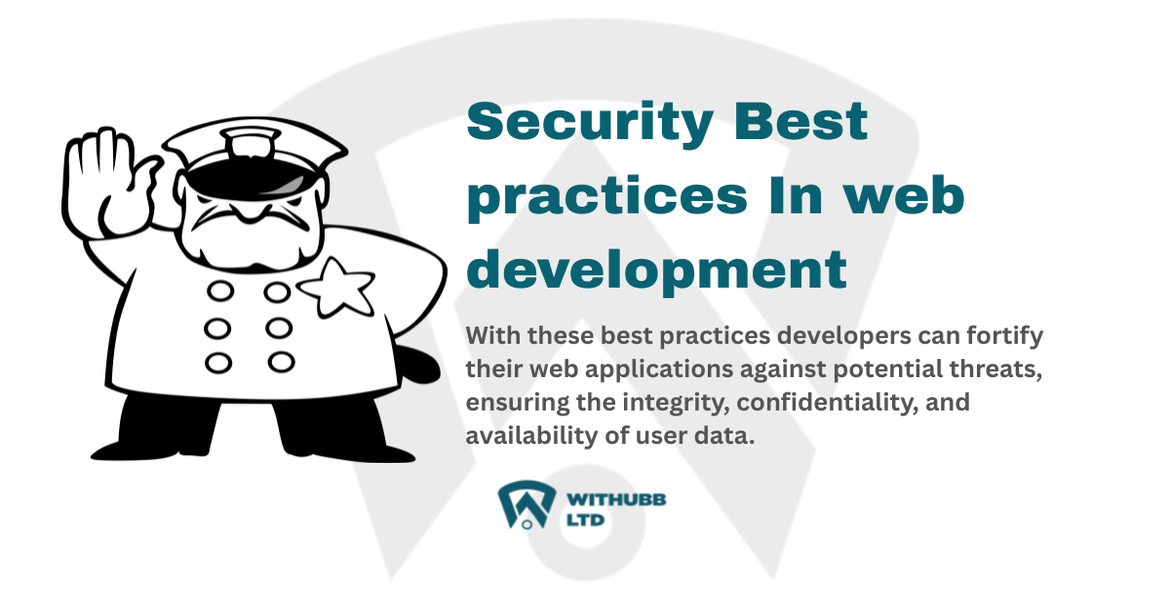
Security Best practices In web development
3 weeks, 2 days ago · 9 min read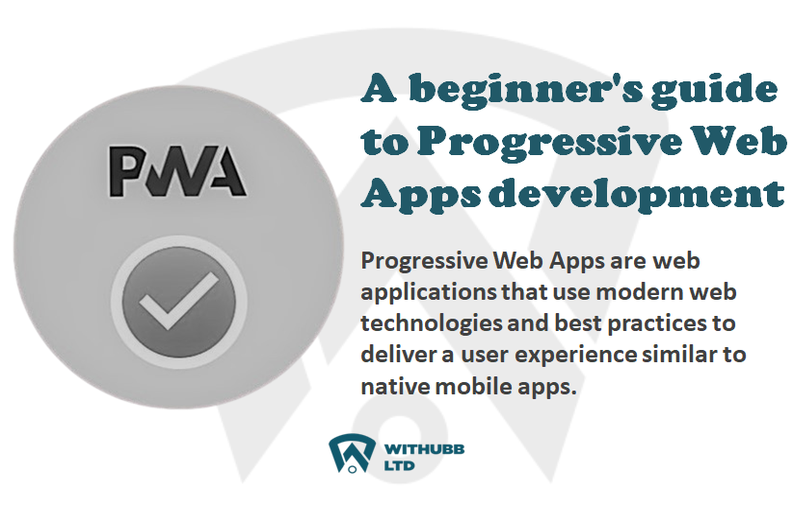
A beginner's guide to Progressive Web Apps development (PWA)
3 weeks, 2 days ago · 5 min read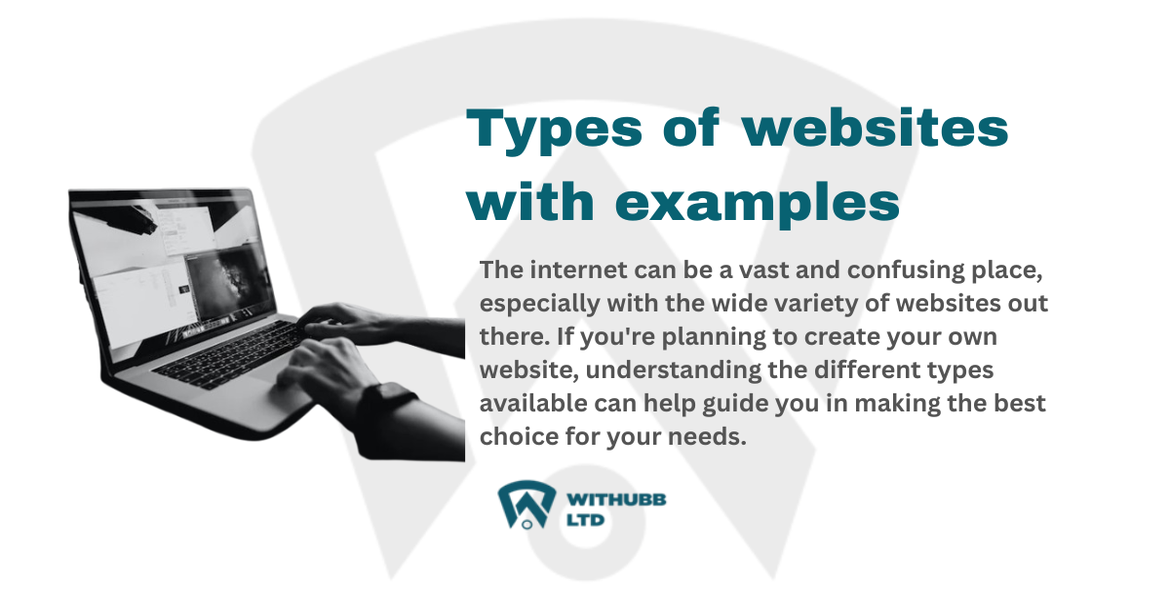
Types of websites with examples
3 weeks, 2 days ago · 10 min read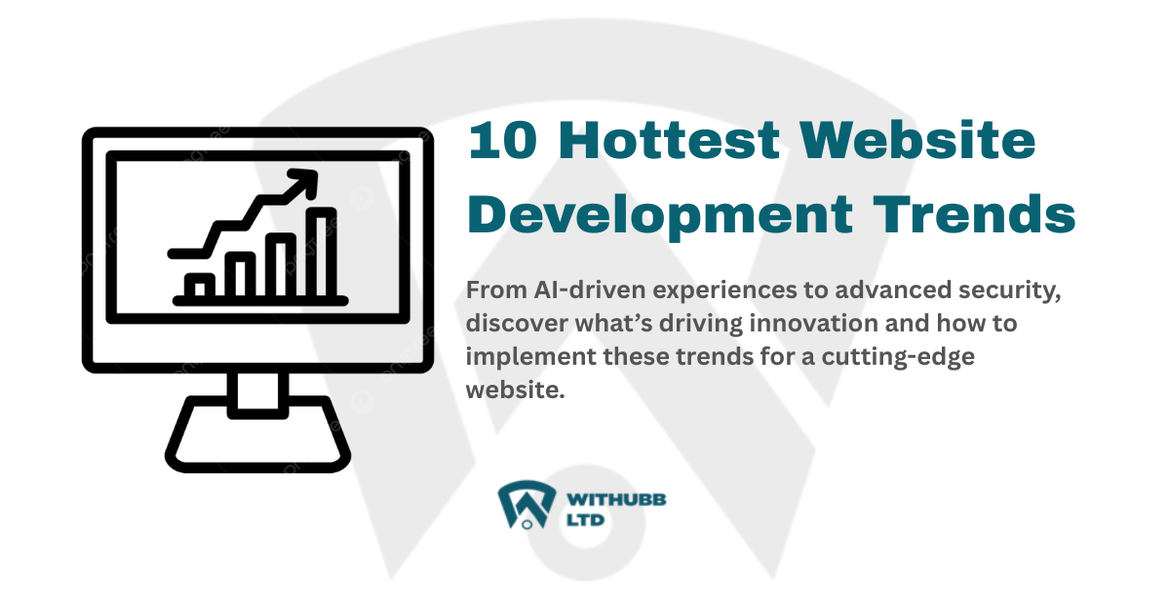
10 Hottest Website Development Trends You Can’t Ignore in 2025
3 weeks, 2 days ago · 10 min read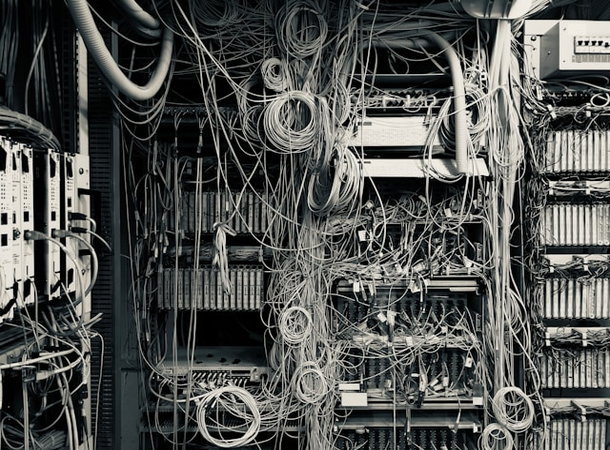
How to Set Up Django with PostgreSQL, Nginx, and Gunicorn on Ubuntu VPS Server
4 weeks ago · 12 min read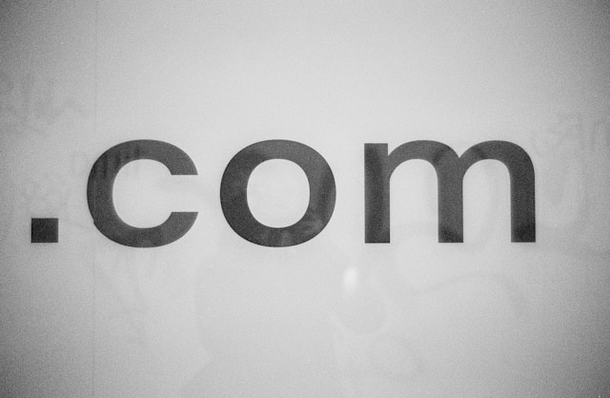
Best Domain Extension Guide: .com vs .net vs .org vs .ng (2025)
4 weeks ago · 6 min read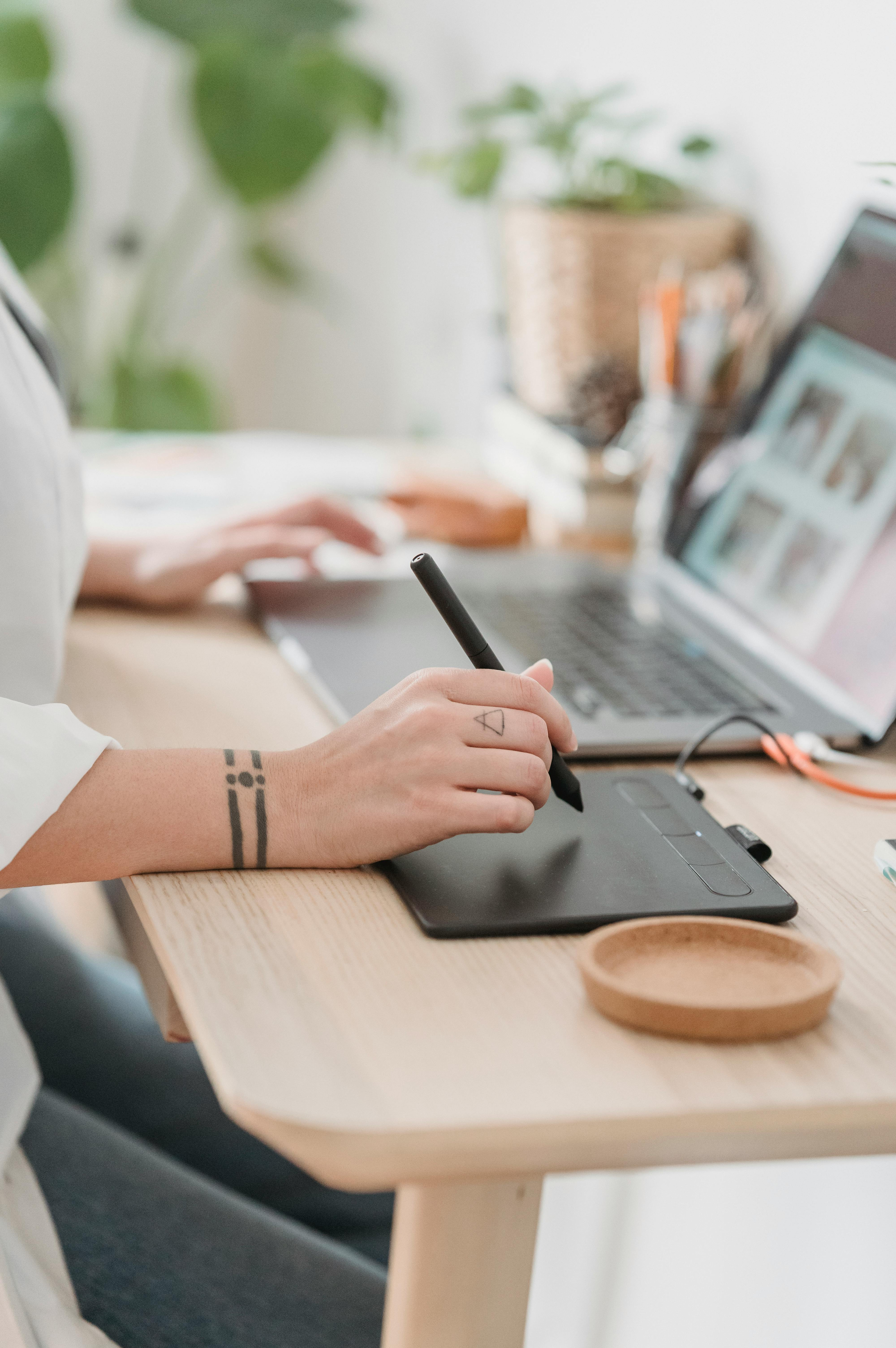
Top Web Design Tools for Professionals in 2025: Best UI/UX & Development Software
4 weeks ago · 6 min read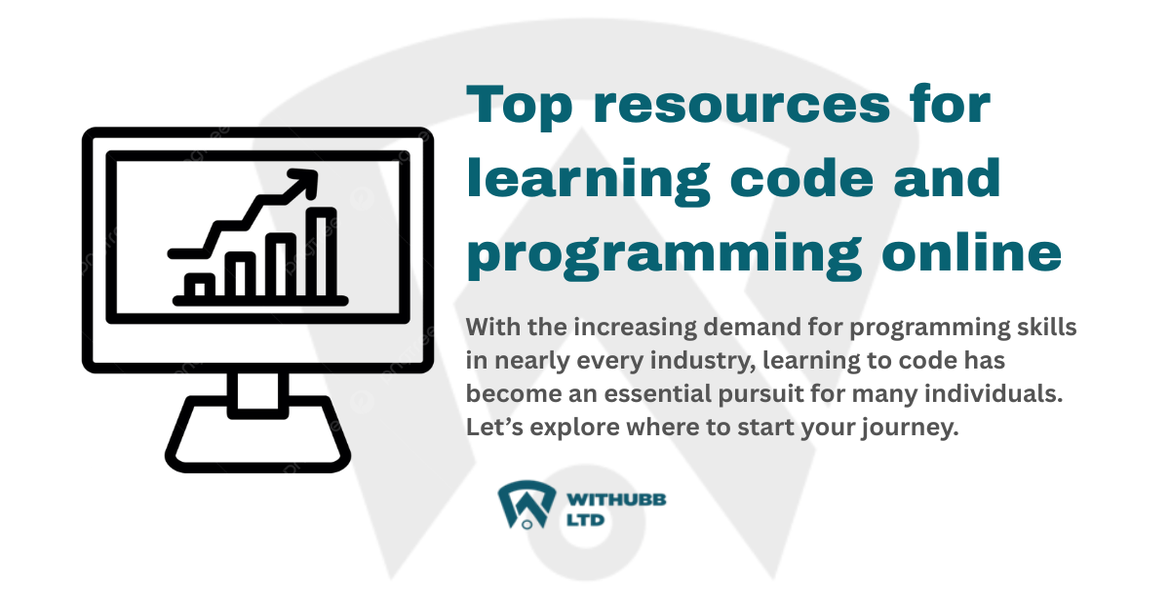
Top 20 resources for learning code and programming online in 2025
4 weeks ago · 7 min read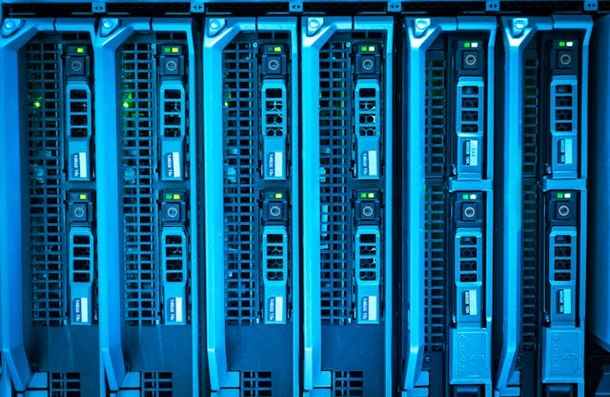
A comprehensive guide to database backup and recovery (Tips, Strategies, Common mistakes)
1 month ago · 12 min read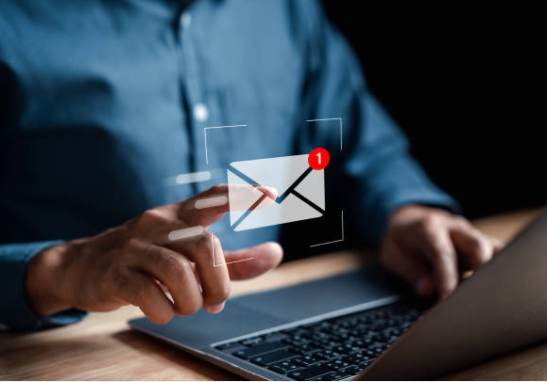
x