What is debugging? How to debug a code for beginners
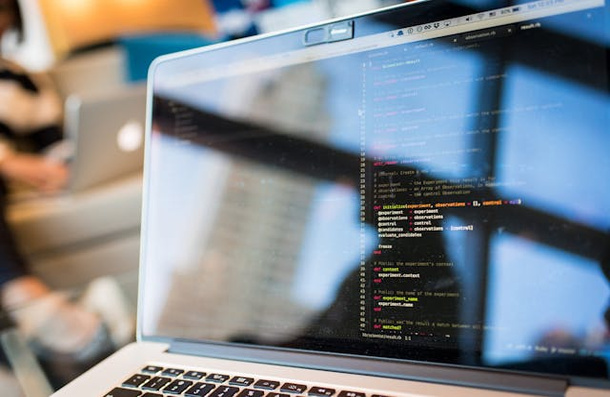
Debugging is a critical aspect of software development that directly impacts the quality, reliability, and performance of applications. It involves identifying, isolating, and fixing issues or "bugs" in a program that cause it to behave unexpectedly. Debugging is not just about making the code run; it’s about making sure the code performs optimally and without error. In this article, we will explore the importance of debugging, how it fits into the development process, and how developers can leverage it to ensure they create robust, reliable software.
What is Debugging?
At its core, debugging is the process of identifying, diagnosing, and resolving errors or bugs in a program. These bugs can arise from various sources such as incorrect logic, syntax mistakes, memory management issues, or runtime errors. Debugging can be done at various stages of development, from the initial writing of code through to post-release maintenance. While it might seem like a simple task on the surface, debugging is a complex and essential skill for developers, ensuring that software runs smoothly and delivers the expected results to users.
Importance of Debugging
The importance of debugging in software development cannot be overstated. Debugging not only helps fix bugs and errors but also plays a key role in improving code quality, optimizing performance, and ensuring the application is secure and scalable. Below are some of the key reasons why debugging is so critical.
1. Ensuring the Functionality of the Application
One of the primary reasons for debugging is to ensure that the application works as expected. Bugs can cause software to malfunction, leading to issues such as crashes, incorrect outputs, or improper user experience. Through debugging, developers can pinpoint the exact source of these problems and fix them, making sure the application meets the expected functionality.
2. Improving Code Quality
Debugging helps improve the overall quality of the codebase. While fixing bugs, developers often refactor and optimize the code. This leads to cleaner, more efficient, and maintainable code. The process of debugging forces developers to carefully examine their code, which often uncovers potential areas for improvement that may have been overlooked during development.
3. Enhancing Performance
Performance optimization is another significant benefit of debugging. By identifying bugs that are causing slowdowns, memory leaks, or excessive resource usage, developers can optimize the performance of their applications. Debugging tools provide insights into memory allocation, CPU usage, and other performance metrics, which can help pinpoint areas where code can be optimized for better efficiency.
4. Preventing Security Vulnerabilities
Bugs in the code can lead to security vulnerabilities, making the application susceptible to hacking, data breaches, or malicious attacks. Debugging is an essential process for identifying and fixing security flaws. By debugging the code thoroughly, developers can ensure that sensitive information is protected and that security risks are minimized.
5. Building a Better User Experience
A smooth, error-free user experience is crucial for retaining users and ensuring customer satisfaction. Debugging plays a vital role in identifying issues that could negatively affect the user experience, such as UI bugs, crashes, or slow load times. By fixing these issues, developers can improve the overall usability and performance of the application, which enhances user satisfaction and trust.
6. Facilitating Collaboration Among Developers
In collaborative projects, debugging helps ensure that multiple developers are on the same page when it comes to the code. By catching bugs early in the development process, debugging prevents miscommunications and reduces the chances of developers inadvertently introducing errors in each other’s work. It promotes clean, understandable code, making it easier for team members to work together.
Understanding the Debugging Process
Debugging is not just a random task but a systematic process that requires a methodical approach. There are several stages in the debugging process, and understanding these stages can help developers debug more effectively.
1. Identifying the Bug
The first step in the debugging process is identifying that a bug exists. This often happens when the software does not perform as expected, crashes, or throws an error. Developers use logs, error messages, or reports from users to understand that something is wrong. Sometimes bugs may be harder to detect, requiring further investigation.
2. Reproducing the Error
Once a bug is identified, the next step is to reproduce it consistently. This step helps developers understand the conditions under which the bug occurs. Reproducing the error may involve retracing specific actions in the code, such as inputting certain values or performing particular tasks. Reproducing the error helps narrow down the cause of the issue.
3. Isolating the Cause
After reproducing the error, developers need to isolate the source of the bug. This is often the most challenging part of debugging because bugs may arise from various areas in the code. Developers often use debugging tools such as breakpoints, step-through execution, and variable inspection to isolate the problematic section of code.
4. Fixing the Bug
Once the bug is isolated, the next step is fixing it. This involves modifying the code, correcting the logic, or adjusting variables to ensure that the issue is resolved. Developers may also need to write additional tests to ensure that the fix does not introduce new issues elsewhere in the application.
5. Testing and Verification
After a bug is fixed, it’s important to thoroughly test the application to ensure that the fix works and that no new bugs have been introduced. This step includes regression testing, where developers test other parts of the application to ensure that the fix did not break anything else. Automated tests, unit tests, and integration tests are commonly used during this phase.
6. Documenting the Fix
Once the bug is fixed and the application is verified, it’s important to document the fix. This documentation helps future developers understand the issue and the resolution, and it provides a reference if the bug reappears. Documentation is particularly helpful in team environments where multiple developers may be working on the same codebase.
Debugging Tools and Software
There are specialized debugging tools that developers can use to assist in the debugging process. These tools vary depending on the programming language and the environment in which the application is being developed. Some of the most popular debugging tools include:
GDB (GNU Debugger)
GDB is a powerful debugger for C/C++ programs. It allows developers to examine what is happening inside the program while it runs and helps identify bugs.
Chrome DevTools
This suite of web developer tools is built into Google Chrome and helps developers debug JavaScript, HTML, and CSS issues in web applications.
Xcode Debugger
Xcode is the integrated development environment (IDE) for macOS and iOS development, and its built-in debugger helps track down bugs in native applications for Apple devices.
Visual Studio Debugger
Visual Studio provides a rich debugging experience for .NET developers, offering breakpoints, step-through debugging, and variable inspection.
Types of Bugs in Programming
Not all bugs are the same. Understanding the different types of bugs that can occur during programming helps developers know how to approach debugging. Some common types of bugs include:
1. Syntax Errors
Syntax errors occur when the code does not follow the rules of the programming language. These errors are easy to spot because they prevent the program from compiling or running. They are typically caused by typos, missing punctuation, or incorrect keywords.
2. Logic Errors
Logic errors happen when the program runs without crashing but produces incorrect results. These bugs are often harder to identify because they do not cause the program to fail outright. Debugging logic errors requires careful analysis of the program's intended behavior and output.
3. Runtime Errors
Runtime errors happen while the program is running and often result in crashes or unexpected behavior. Common runtime errors include dividing by zero, referencing null values, or accessing array elements out of bounds. These errors can often be detected through careful testing and logging.
4. Memory Leaks
Memory leaks occur when the program does not properly release memory that is no longer needed. This causes the program to consume more memory over time, potentially slowing down or crashing. Memory leaks are most common in languages like C and C++ that require manual memory management.
5. Race Conditions
Race conditions happen in multithreaded programs when two or more threads access shared resources simultaneously, leading to unpredictable behavior. Debugging race conditions can be particularly challenging because the bug may not always occur in a predictable manner. Specialized tools are often needed to detect and resolve these issues.
Best Practices for Effective Debugging
To be more efficient in debugging, developers should adhere to some best practices that can help streamline the process and ensure better code quality. These best practices include:
1. Keep the Code Simple and Clean
Writing clean and simple code makes it easier to debug. Complex code with convoluted logic can obscure bugs and make it harder to identify issues. Keeping the code clean ensures that developers can easily track down problems when they arise.
2. Write Tests
Writing unit tests and integration tests helps catch bugs early and ensures that the code works as expected. Tests can also serve as a safeguard, ensuring that bug fixes do not inadvertently introduce new errors.
3. Break the Problem Down
If a bug seems difficult to fix, break it down into smaller, manageable pieces. Isolating sections of code or limiting the scope of the problem can make debugging more efficient.
4. Use Pair Programming
Pair programming involves two developers working together on the same task. One writes the code while the other reviews it in real time. This collaboration often leads to faster identification of bugs and higher-quality code.
5. Don’t Overlook the Basics
Sometimes, bugs can be traced back to basic issues like incorrect file paths or missing dependencies. Always double-check the basics before diving into complex debugging techniques.
Conclusion
Debugging is an essential skill for any developer. It ensures that applications are functional, reliable, and meet the expectations of end users. While the debugging process can sometimes feel tedious, understanding its importance and following best practices can make the task more manageable. By investing time in mastering debugging techniques and using the right tools, developers can ensure the robustness of their applications and deliver high-quality software that performs well in the real world. Debugging is not just about fixing errors; it's about creating a culture of continuous improvement and producing reliable, scalable code.
Want to Build Something Amazing?
We prioritize your business success and we deliver faster. Our services are custom and build for scale.
Start nowMore Articles
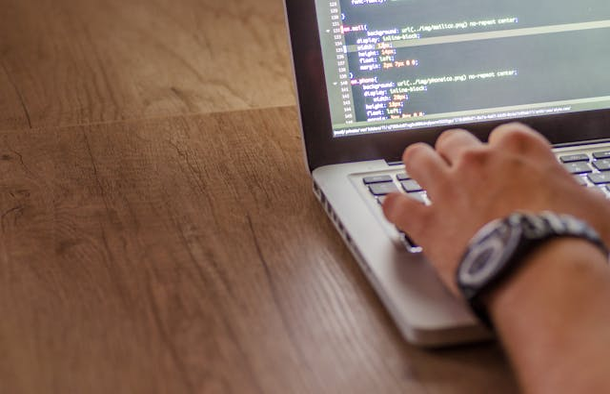
Object-Oriented Programming (OOP) Meaning, Principles, Benefits.
1 week, 1 day ago · 7 min read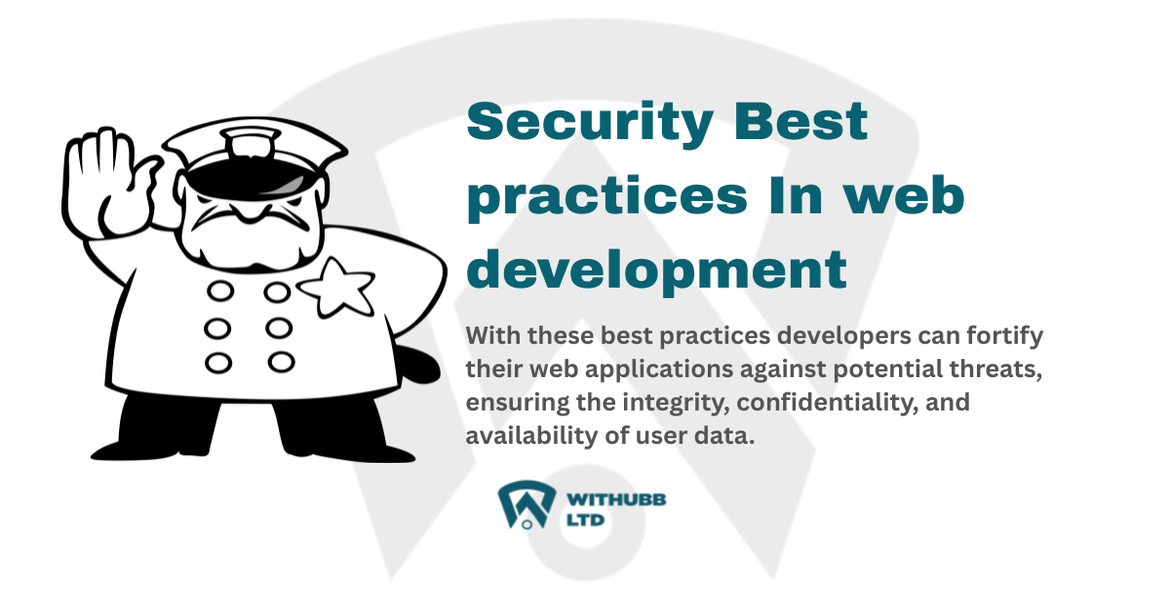
Security Best practices In web development
3 weeks, 2 days ago · 9 min read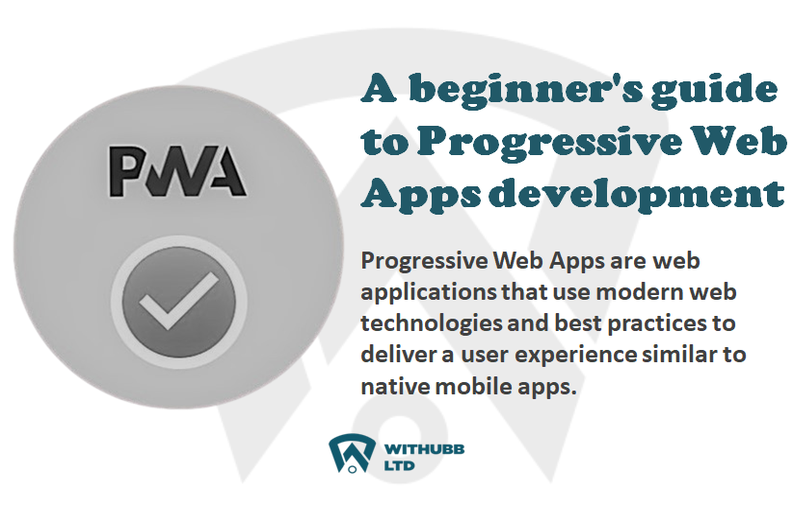
A beginner's guide to Progressive Web Apps development (PWA)
3 weeks, 2 days ago · 5 min read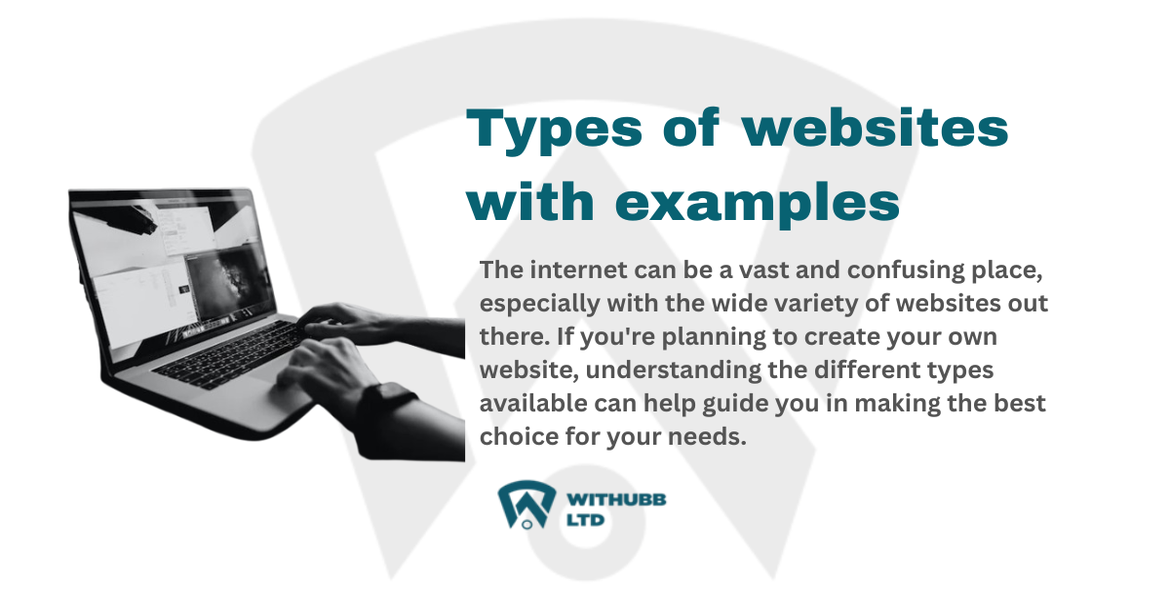
Types of websites with examples
3 weeks, 2 days ago · 10 min read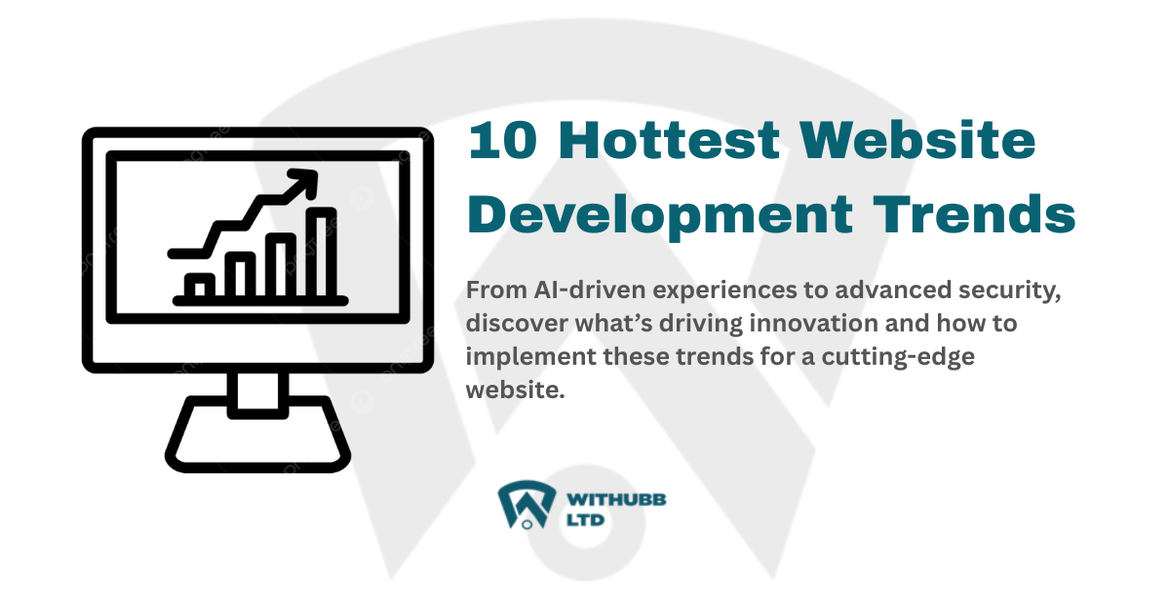
10 Hottest Website Development Trends You Can’t Ignore in 2025
3 weeks, 2 days ago · 10 min read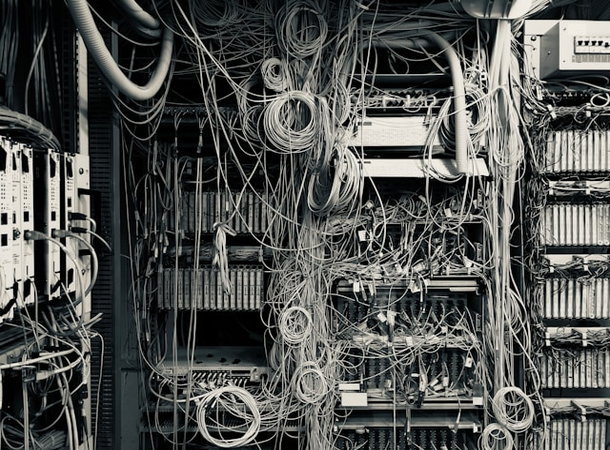
How to Set Up Django with PostgreSQL, Nginx, and Gunicorn on Ubuntu VPS Server
4 weeks ago · 12 min read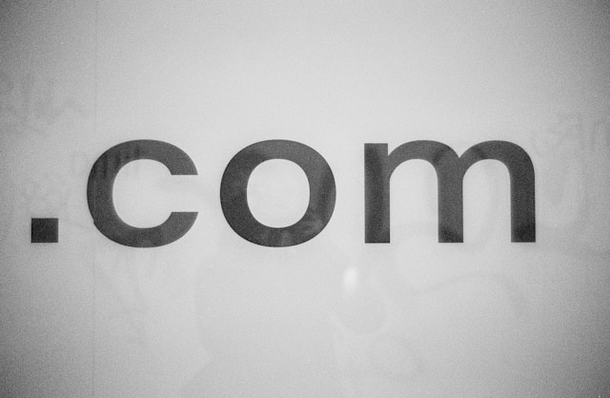
Best Domain Extension Guide: .com vs .net vs .org vs .ng (2025)
4 weeks ago · 6 min read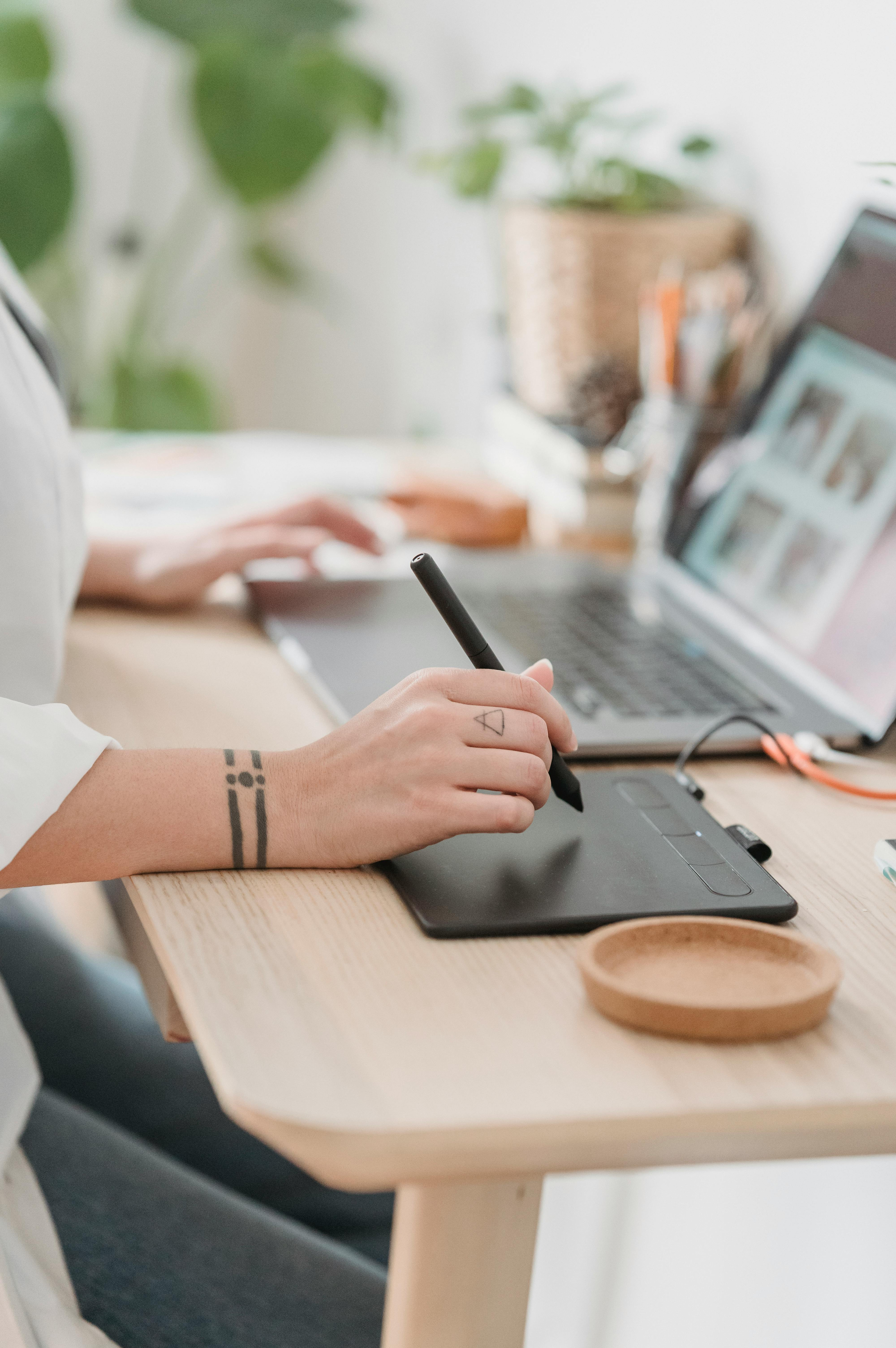
Top Web Design Tools for Professionals in 2025: Best UI/UX & Development Software
4 weeks ago · 6 min read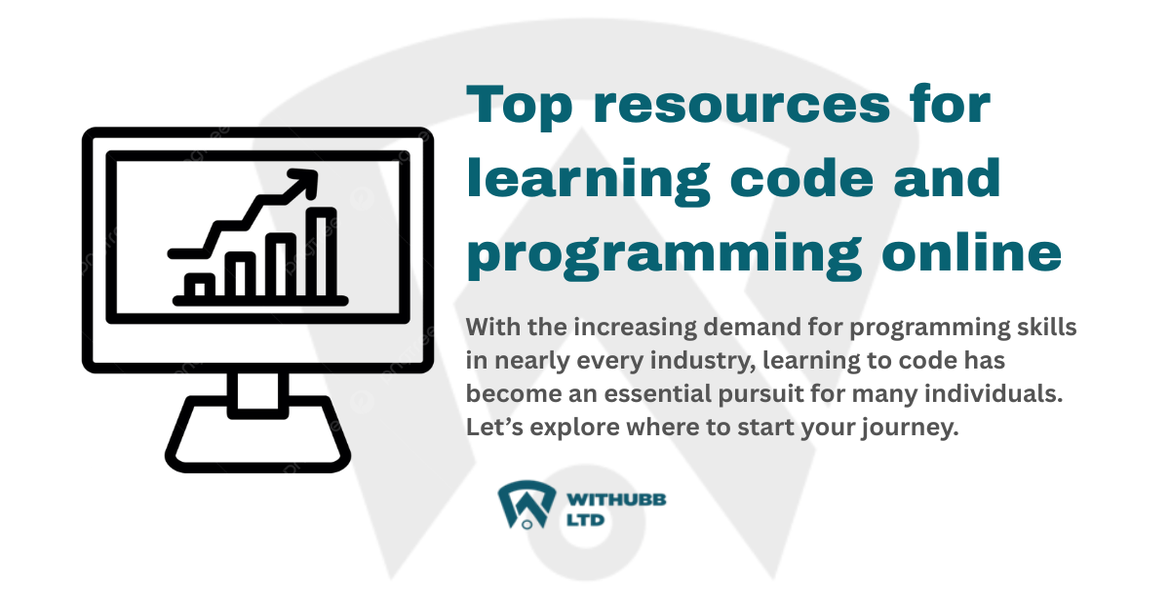
Top 20 resources for learning code and programming online in 2025
4 weeks ago · 7 min read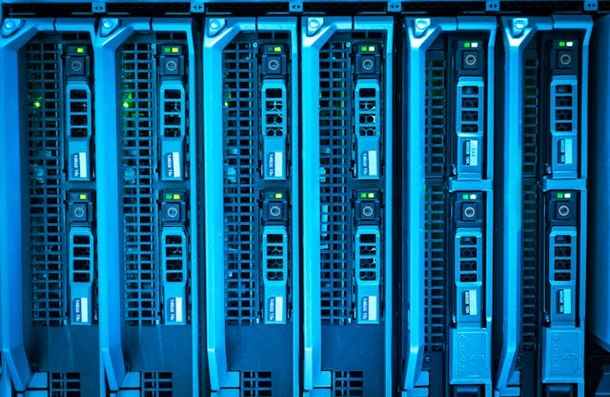
A comprehensive guide to database backup and recovery (Tips, Strategies, Common mistakes)
1 month ago · 12 min read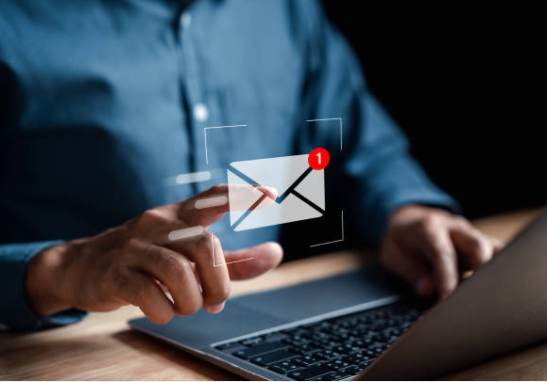
x